Here I’ll start a series of little post that will build a new application based on the following stack: ASP.NET MVC5, Angular 1.5.x and MongoDB.
The code used in these tutorials can be found under my git hub project: myClub
Every post has his own dedicated branch, so you can just use “git checkout <branchname>” to find all the source code of the post you’re interesting in.
1.0 Create a new MVC core project
To generate a new project we’ll use Yeomen. Open a commend prompt/bash shell or powershell shell on the location you want to put your new project.
mkdir myClub
cd myClub
yo aspnet
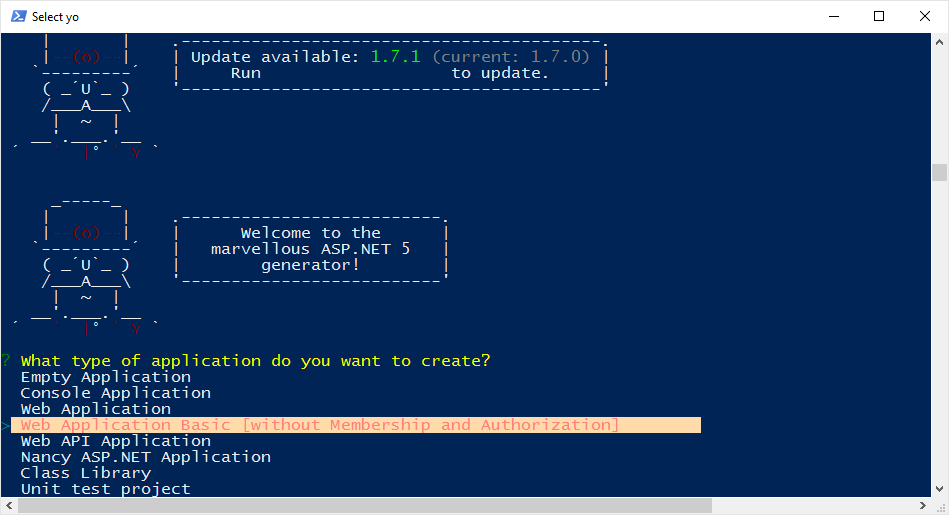
Choose Web Application Basic
Provide a name.
1.1. Add Angular to MVC app
1) Add Angular, from command line inside you project dir:
bower install angular angular-ui-router –save
Add angular to _layout.cshtml :
<script src="~/lib/angular/angular.min.js"></script>
2) Create two js scripts under wwwroot
app.config.js
(function () {
'use strict';
angular.module('myClub')
.config(initrouter);
function initrouter($stateProvider, $locationProvider, $urlRouterProvider) {
$locationProvider.html5Mode(true);
$urlRouterProvider.otherwise('/');
$stateProvider
.state(
'home',
{
url: '/',
templateUrl: 'app/home.html',
controller: "HomeController",
controllerAs: 'vm'
}
)
.state(
'myteam',
{
url: '/myteam',
templateUrl: 'app/myTeam.html',
controller: "MyTeamController",
controllerAs: 'vm'
}
);
}
})();
app.module.js
(function() {
'use strict';
angular.module('myClub', ['ui.router']);
})();
3) Add two controllers
myTeam.controller.js
(function () {
'use strict';
angular
.module('myClub')
.controller('HomeController', myTeam);
myTeam.$inject = ['$location'];
function myTeam($location) {
/* jshint validthis:true */
var vm = this;
vm.players = [];
vm.title = 'Home';
activate();
function activate() {
}
}
})();
home.controller.js
(function () {
'use strict';
angular
.module('myClub')
.controller('HomeController', myTeam);
myTeam.$inject = ['$location'];
function myTeam($location) {
/* jshint validthis:true */
var vm = this;
vm.players = [];
vm.title = 'Home';
activate();
function activate() {
}
}
})();
4) Add two html pages
home.html
<div class="jumbotron">
<div class="container">
<h1>MyClub</h1>
<p></p>
</div>
</div>
<div>
</div>
myteam.html
<div>
<p>My Team</p>
</div>
5) Reference the js scripts in _layout.cshtml
<script src="~/lib/angular/angular.min.js"></script>
<script src="~/lib/angular-ui-router/release/angular-ui-router.js"></script>
<script src="~/app/app.module.js"></script>
<script src="~/app/app.config.js"></script>
<script src="~/app/home.controller.js"></script>
<script src="~/app/myTeam.controller.js"></script>
6) Add angular app inside and move the navbar from _layout.html to index.cshtml
Index.cshtml